- Published on
Mac setup for Developer
- Authors
- Name
- Do Dinh Si
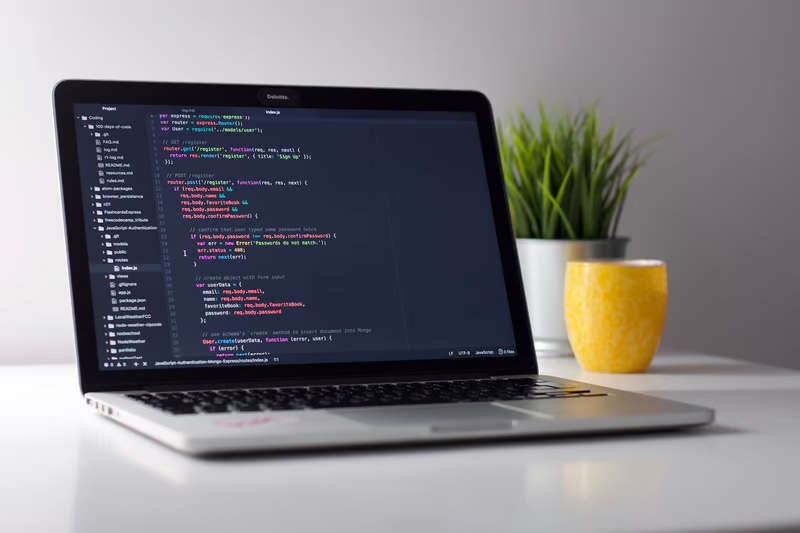
After using Macbook for a few years. Here are some essential settings for programming that I find helpful. It saves me time searching when I have to reinstall my Mac.
System preferences
Override more system preferences from the terminal:
# take screenshots as jpg (usually smaller size) and not png
defaults write com.apple.screencapture type jpg
# change screenshot destination (desktop works fine also)
defaults write com.apple.screencapture location "~/Screenshots"
# do not open previous previewed files (e.g. PDFs) when opening a new one
defaults write com.apple.Preview ApplePersistenceIgnoreState YES
# show Library folder
chflags nohidden ~/Library
# show hidden files
defaults write com.apple.finder AppleShowAllFiles YES
# show path bar
defaults write com.apple.finder ShowPathbar -bool true
# show status bar
defaults write com.apple.finder ShowStatusBar -bool true
killall Finder;
Xcode and Command Line Tools
You must install Xcode and Command Line Tools before proceeding with the rest of the guide.
To install Xcode: Open App Store, install Xcode. It's a large download and will take a while.
After the Xcode installation finishes, go to install Command Line Tools
. Open the terminal app and run:
xcode-select --install && sudo xcodebuild -license
Homebrew
Homebrew is a package manager trusted by many people. To install, open Terminal or iTerm and run the command:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
After installing, you can run brew doctor
to check your system for potential problems. And run brew update
to update everything to recent version.
To search for a package
brew search [keyword]
To install a formulae
brew install [package name]
To install a cask
brew install --cask [package name]
Other helpful commands
# Open Homebrew home page
brew home
# List all installed formulae/cask
brew list
brew list --cask
# Uninstall a package
brew uninstall [package name]
brew uninstall -cask [package name]
# Search package with description
brew search [keyword] --desc
# Update brew package
brew update
# Show outdated package
brew outdated
# Upgrade installed package
brew upgrade [package name]
# Upgrade all installed package
brew upgrade
# Remove unused package
brew cleanup
Other helpful commands for service
# List all services
brew services list
# Start a service
brew services start [service name]
# Stop a service
brew services stop [service name]
# Restart a service
brew services restart [service name]
# Remove unused services
brew services cleanup
iTerm 2
This is my preferred terminal setup. Feel free to use whatever you like.
To install iTerm 2. Open Terminal and run the command:
brew install --cask iterm2
iTerm preferences
- Natural Text Editing: Turn on Natural Text Editing by
Preferences -> Profiles -> Keys -> Select Presets -> Natural Text Editing
. This allows you to use the Options/Command keys to move your cursor freely. - Font: Change the font of the terminal by
Preferences -> Profiles -> Text
. I use hack-nerd-font with 14pt font size. This font can be install from Homebrew with commandbrew install --cask font-hack-nerd-font
. - Default editor: Change the default editor when open from Terminal by
Preferences -> Profile -> Advanced -> Semantic History -> Open with Editor -> VS Code
.
Oh my zsh
Oh My Zsh using for managing your Zsh configuration. Run the following command to install:
sh -c "$(curl -fsSL https://raw.githubusercontent.com/ohmyzsh/ohmyzsh/master/tools/install.sh)"
Some commonly used Oh My Zsh plugins are:
Zsh theme
After that, you can install the Zsh theme to personalize your command line window. There are some popular themes as the following:
Git
Git is already installed as part of Xcode Command Line Tools. However, I prefer to install the latest version from brew and avoid using the outdated version installed by Apple.
brew install git
Git global configurations
After installing Git, apply the following global configurations:
Set up the default branch for any new project to main (This was introduced in Git version 2.28):
git config --global init.defaultBranch main
Enable colors in command output:
git config --global color.uni auto
Improved git log:
git config --global alias.lg "log --color --graph --pretty=format:'%Cred%h%Creset -%C(yellow)%d%Creset %s %Cgreen(%cr) %C(bold blue)<%an>%Creset' --abbrev-commit"
Now use:
git lg
Set up real name and email:
git config --global user.name "Real name"
git config --global user.email email@domain.com
To check or verify the global git config, run:
git config -l --global
Setup SSH keys for multiple account
Step 1: Generate and add SSH key for all account as github official document link bellow:
Step 2: Create config
file in ~/.ssh
directory (if not exist) and add host for multiple account to config host entries:
#sidd-office account
Host github.com-sidd-office
HostName github.com
User git
IdentityFile ~/.ssh/github-sidd-office
#sidd-personal account
Host github.com-sidd-personal
HostName github.com
User git
IdentityFile ~/.ssh/github-sidd-personal
Step 3: Clone the repository use the bellow command:
git clone git@github.com-{your-username}:{owner-user-name}/{the-repo-name}.git
[e.g.] git clone git@github.com-sidd-personal:sidd88/TestRepo.git
And finally, config user.name
, user.email
for local repository corresponding with github account.
Versions management
There are various package managers for different programming languages and technology stacks. Here are the environment managers I frequently use:
jEnv
Before using jEnv, we must install Java. Run the following command to install Java from Homebrew
:
brew install openjdk
For the system Java wrappers to find this JDK, symlink it with:
sudo ln -sfn /usr/local/opt/openjdk/libexec/openjdk.jdk /Library/Java/JavaVirtualMachines/openjdk.jdk
To check Java environments installed:
/usr/libexec/java_home -V
After installing an OpenJDK version, add them to jenv
:
jenv add <jdk path>
You can show and copy jdk path by/usr/libexec/java_home -V
command. Example as bellow
Commonly jenv
used commands:
# Lis versions added
jenv versions
# Set Java version for the current working directory
jenv local [version]
# Delete local java version
jenv local --unset
jenv
can set Java versions at 3 levels:
- global (lowest priority)
- local
- shell (highest priority)
To have JAVA_HOME get set by jEnv, enable the export plugin.
eval "$(jenv init -)"
jenv enable-plugin export
goenv
Go version management. To install Goenv, run following command:
brew install goenv
To show all available Go versions:
goenv install -l
To install a go version:
goenv install <version>
Zsh
configured for goenv
:
export GOENV_ROOT="$HOME/.goenv"
export PATH="$GOENV_ROOT/bin:$PATH"
eval "$(goenv init -)"
export PATH="$GOROOT/bin:$PATH"
export PATH="$PATH:$GOPATH/bin"
Commonly goenv
used commands:
# Set Go version for the current working directory
goenv local [version]
# Set Go version for global
goenv global [version]
# Lists all available goenv commands.
goenv commands
nvm
Manage multiple Node.js versions. To install nvm, run the following command:
brew install nvm
Add bellow script to ~/.zshrc:
export NVM_DIR="$([ -z "${XDG_CONFIG_HOME-}" ] && printf %s "${HOME}/.nvm" || printf %s "${XDG_CONFIG_HOME}/nvm")"
[ -s "$NVM_DIR/nvm.sh" ] && \. "$NVM_DIR/nvm.sh" # This loads nvm
To install latest node version:
nvm install --lts
To use latest Node
version:
nvm use --lts
To install a specific version of Node
:
nvm intall <version>
To use a specific Node
version:
nvm use <version>
Use nvm alias default node
to make the latest version the default.
Run nvm --help
to show how to use other commands.
Alias
Some aliases for some of the shell commands that I frequently use:
# Zsh
alias zcon="code ~/.zshrc"
alias zsrc="source ~/.zshrc"
# Global config
alias sshcon="code ~/.ssh/config"
alias gitcon="vim ~/.gitconfig"
# Git
alias gits="git status"
alias gitd="git diff"
alias gitl="git lg"
alias gita="git add ."
alias gitc="git commit"
# List all available iOS simulators
alias listios="xcrun simctl list devices"